Table Of Content
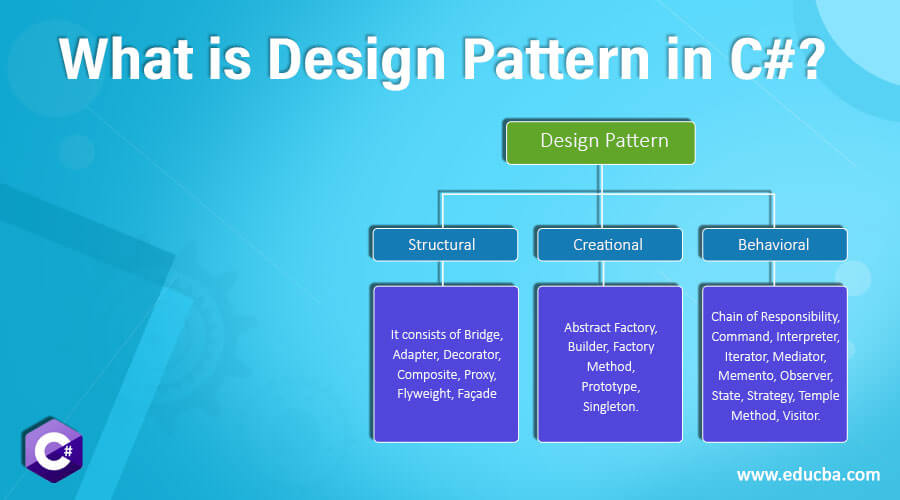
To add a new product type, one could easily introduce a new factory method without altering the existing factories. This flexibility ensures that the factory pattern remains one of the most widely used patterns in software design. While C doesn't have the same object-oriented capabilities as some languages, it can still benefit from many design patterns. These patterns offer structured and efficient solutions to recurring coding problems. Here, we'll explore some of the most prevalent patterns and how they can be implemented in C. Structural class-creation patterns use inheritance to compose interfaces.
Interpreter Method Design Pattern
Software design patterns are communicating objects and classes that are customized to solve a general design problem in a particular context. Software design patterns are general, reusable solutions to common problems that arise during the design and development of software. They represent best practices for solving certain types of problems and provide a way for developers to communicate about effective design solutions. It achieves this by creating a set of decorator classes that are used to wrap concrete components, which represent the core functionality. Developers use patterns for their specific designs to solve their problems. Pattern choice and usage among various design patterns depend on individual needs and concerns.
Disadvantages of the Decorator Pattern in C++ Design Patterns
Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. Lets you compose objects into tree structures and then work with these structures as if they were individual objects. Lets you produce families of related objects without specifying their concrete classes.

Classification
The XMLToJSONAdapter class is used to convert the XML data from EmployeeAPI into JSON format, making it compatible with the rest of the system that expects JSON data. You have a web-to-print onlineshop and want to add high quality content? Pattern Design is a stock platform for buying and selling high-quality seamless vector patterns. A Pascal’s Triangle is a triangular array of binomial coefficients where the nth row contains the binomial coefficients nC0, nC1, nC2, …….
The Strategy pattern is based on the idea of encapsulating a family of algorithms into separate classes that implement a common interface. Behavioral design patterns are a subsetof design patterns in software development that deal with the communication and interaction between objects and classes. They focus on how objects and classes collaborate and communicate to accomplish tasks and responsibilities. The Adapter Design Pattern is useful for ensuring compatibility between classes with incompatible interfaces. It provides greater flexibility and reusability of existing code, making it simpler to integrate new classes with old code.
thoughts on “Design Patterns in C# With Real-Time Examples”
Before starting with design patterns in .NET, let's understand the meaning of design patterns and why they are useful in software architecture and programming. The Model-View-Controller paradigm is touted as an example of a "pattern" which predates the concept of "design patterns" by several years. These design patterns are all about Class's objects communication. Behavioral patterns are those patterns that are most specifically concerned with communication between objects. You can use one design pattern or a combination of design patterns to solve a problem in your software application.
The third form, .NET optimized, demonstrates design patterns that fully exploit the latest C# and .NET features, such as, generics, reflection, lambdas, primary constructors, and more. Singleton Method is a creational design pattern, it provide a class has only one instance, and that instance provides a global point of access to it. According to the book "Design Patterns, Elements of Reusable Object-Oriented Software," the Adapter design pattern converts the interface of a class into another interface clients expect. The adapter lets classes work together that couldn't otherwise because of incompatible interfaces. The world of C programming is expansive, and design patterns act as guiding beacons for developers, aiding them in writing efficient and maintainable code. Let's delve into the benefits of incorporating these design patterns into your C projects.

Modernes C++ Mentoring
While Singleton provides an organized way of accessing a single instance, it comes with its set of challenges in a multi-threaded environment. It's essential to ensure thread safety, usually using mutexes, to prevent potential race conditions during instantiation. Design patterns encourage reusability by promoting standardized coding practices. They are tested solutions to frequent challenges, enabling you to reuse them across multiple projects. By understanding and applying such patterns, you not only develop efficient code but also ensure your code stands the test of time.
In this article, we learn and understand Creational Design Patterns in detail, including a UML diagram, template source code, and a real-world example in C#. Creational Design Patterns provide ways to instantiate a single object or group of related objects. These patterns deal with the object creation process in such a way that they are separated from their implementing system. That provides more flexibility in deciding which object needs to be created or instantiated for a given scenario. Creational design patterns solve the problems related to object creation.
Evaluate if a pattern truly addresses the problem at hand or if a simpler solution exists. As the system evolves, adding more observers or even new types of subjects is straightforward. The decoupled nature ensures minimal disruption and maximizes maintainability. At the heart of the Observer Pattern is a clear separation of concerns. Subjects contain the core business logic, while observers handle the auxiliary functions that respond to changes in the subject. The Observer Pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
These are the basic objects or classes that implement the Component interface. They are the objects to which we want to add new behavior or responsibilities. In my next post, I start my journey through the “Design Patterns and Architectural Patterns with C++”.
Software design patterns classification and selection using text categorization approach - ScienceDirect.com
Software design patterns classification and selection using text categorization approach.
Posted: Fri, 11 Oct 2019 09:57:47 GMT [source]
Decorators are responsible for adding new behaviors to the wrapped Component object. Often, they can be combined for more powerful and flexible results. For instance, you might merge Factory and Observer Patterns to produce objects that immediately register themselves as observers. To implement the Observer Pattern in C, structures and function pointers can be used to emulate the interaction between subjects and observers. To understand better, let's implement a factory for creating different types of products.
As we progress in this Design Patterns series, you will understand what Object Generation and Integration problems are and how we solve them using different design patterns. Design patterns are solutions to software design problems you find again and again in real-world application development. Proxy Method is a structural design pattern, it provide to create a substitute for an object, which can act as an intermediary or control access to the real object.
A google search for "object-oriented C" also yields a number of other good examples and resources. Null Object Method is a Behavioral Design Pattern, it is used to handle the absence of a valid object by providing an object that does nothing or provides default behavior. State Method is a Behavioral Design Pattern, it allows an object to alter its behavior when its internal state changes. Iterator Method is a Behavioral Design Pattern, it provides a way to access elements of an aggregate object (a collection) sequentially without exposing the underlying representation of that collection.
Often, people only understand how to apply certain software design techniques to certain problems. These techniques are difficult to apply to a broader range of problems. Design patterns provide general solutions, documented in a format that doesn't require specifics tied to a particular problem. In software engineering, a design pattern is a general repeatable solution to a commonly occurring problem in software design. A design pattern isn't a finished design that can be transformed directly into code.
No comments:
Post a Comment